Exploring Entity Framework Part 1: A Brief Overview
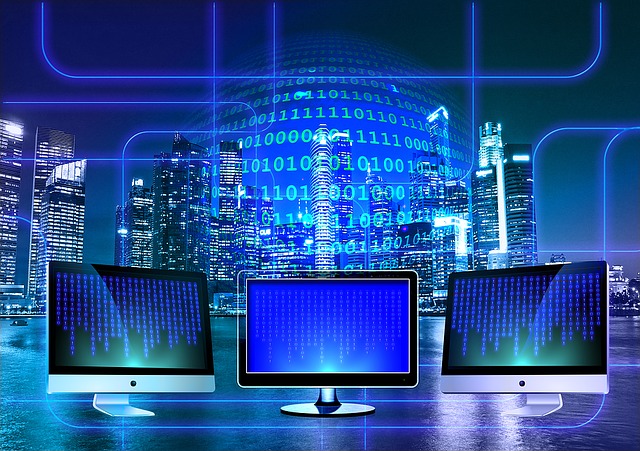
- A means to automatically generate models based on our database architecture.
- The flexibility to tweak the auto-generated code so that we can ensure our classes are using the correct data types.
- The ability to modify models attributes that do not match what we have in our database.